A control structure is a logical design that controls order in which set of statements execute.
A sequence structure is a set of statements that execute in the order they appear.
A decision structure is the specific action(s) performed only if a condition exists and is also known as a selection structure.
In a flowchart, diamonds are used to represents true/false conditions that must be tested. Actions can be conditionally executed and are performed only when a condition is true.
A single alternative decision structure provides only one alternative path of execution. If the condition is not true, exit the structure.
Python syntax:
The first line is known as the if clause and includes the keyword if followed by the condition. The condition can be true or false. When the if statement executes, the condition is tested, and if it is true the block statements are executed. otherwise, the block statements are skipped.
Boolean Expressions and Relational Operators
A boolean expression is tested by if statement to determine if it is true or false.
Example: a > b
This statement is true if a is greater than b; otherwise, it is false.
Relational operators determine whether a specific relationship exists between two values.
Example: greater than (>)
>= and <= operators test more than one relationship. It is enough for one of the relationships to exist for the expression to be true.
== operator determines whether the two operands are equal to one another. Not to be confused with assignment operator (=).
!= operator determines whether the two operands are not equal.
Expression | Meaning |
---|---|
x > y x < y x >= y x <= y x = = y |
Is x greater than y? Is x lesser than y? Is x greater than or equal to y? Is x less than or equal to y? Is x equal to y? |
Any relational operator can be used in a decision block.
Example: if balance == 0
Example: if payment != balance
It is possible to have a block inside another block.
Example: if statement inside a function
Statements in inner block must be indented with respect to the outer block.
if-else Statement
Dual alternative decision structures have two possible paths of execution. One is taken if the condition is true, and the other if the condition is false.
Syntax:
The if clause and else clause must be aligned, and statements must be consistently indented.
A decision structure can be nested inside another decision structure. This is commonly needed in programs.
For example:
To determine if someone qualifies for a loan, they must meet two conditions:
- Must earn at least $30,000/year
- Must have been employed for at least two years
Check the first condition, and if it is true, check the second condition.
It is important to use proper indentation in a nested decision structure. it is Important for the Python interpreter and makes code more readable for programmers.
Rules for writing nested if statements:
- else clause should align with matching if clause
- Statements in each block must be consistently indented
if-elif-else Statement
if-elif-else statement is a special version of a decision structure that makes logic of nested decision structures simpler to write.
Syntax:
Insert as many elif clauses as necessary.
if, elif, and else clauses are all aligned and conditionally executed blocks are consistently indented. if-elif-else statements are never required, but their logic easier to follow. They can be accomplished by nested if-else however, code can become complex, and indentation can cause problematic long lines.
Strings can be compared using the == and != operators. String comparisons are case sensitive. Strings can also be compared using >, <, >=, and <=, Compared character by character based on the ASCII values for each character. If a shorter word is a substring of a longer word, the longer word is greater than the shorter word
Repetition Structures
In programming we often have to write code that performs the same task multiple times. Some of the disadvantages to duplicating code include;
- It makes program large
- It's time consuming
- The code may need to be corrected in many places.
Repetition structures make the computer repeat included code as necessary. This includes condition-controlled loops and count-controlled loops.
Nested Loops
A Nested loop is a loop that is contained inside another loop. for example, an analog clock works like a nested loop. the hour hand moves once for every twelve movements of the minutes hand: for each iteration of the “hours,” do twelve iterations of “minutes”. The Second hand moves 60 times for each movement of the minutes hand: for each iteration of “minutes,” do 60 iterations of “seconds”.
Key points about nested loops:
- Inner loop goes through all of its iterations for each iteration of outer loop
- Inner loops complete their iterations faster than outer loops
- Total number of iterations in nested loop:
A for loop is a Count-Controlled loop that iterates a specific number of times.
They are designed to work with sequence of data items, iterating once for each item in the sequence.
Syntax:
the target variable is the variable which is the target of the assignment at the beginning of each iteration.
The range function simplifies the process of writing a for loop.
range returns an iterable object that contains a sequence of values that can be iterated over.
range characteristics:
- One argument: used as ending limit
- Two arguments: starting value and ending limit
- Three arguments: third argument is step value
The range function can be used to generate a sequence with numbers in descending order. Make sure starting number is larger than end limit, and step value is negative.
Example: range (10, 0, -1)
A while loop is a condition-controlled loop that runs while a condition is true.
While loops have two parts:
- A condition that is tested for true or false value
- Statements that are repeated if the condition is true
In flow chart, a line goes back to previous part.
Syntax:
For a loop to stop executing, something must happen inside the loop to make the condition false.
Iteration is one execution of the body of a loop.
A while loop is known as a pre-test loop because it tests a condition before performing an iteration and will never execute if the condition is false to start with.
Loops must contain within themselves a way to terminate. Something inside a while loop must eventually make the condition false.
An infinite loop occurs when a loop does not have a way of stopping and repeats until program is interrupted. This occurs when the programmer forgets to include stopping code in the loop.
Computer cannot tell the difference between good data and bad data. If the user provides bad input, the program will produce bad output, this is referred to as GIGO (Garbage In, Garbage Out). It is important to design program so that bad input is never accepted.
Input validation is inspecting input before it is processed by the program. If the input is invalid, prompt the user to enter the correct one.
This is commonly accomplished using a while loop which repeats as long as the input is bad.
If the input is bad, display an error message and receive another set of data.
If the input is good, continue to process the input.
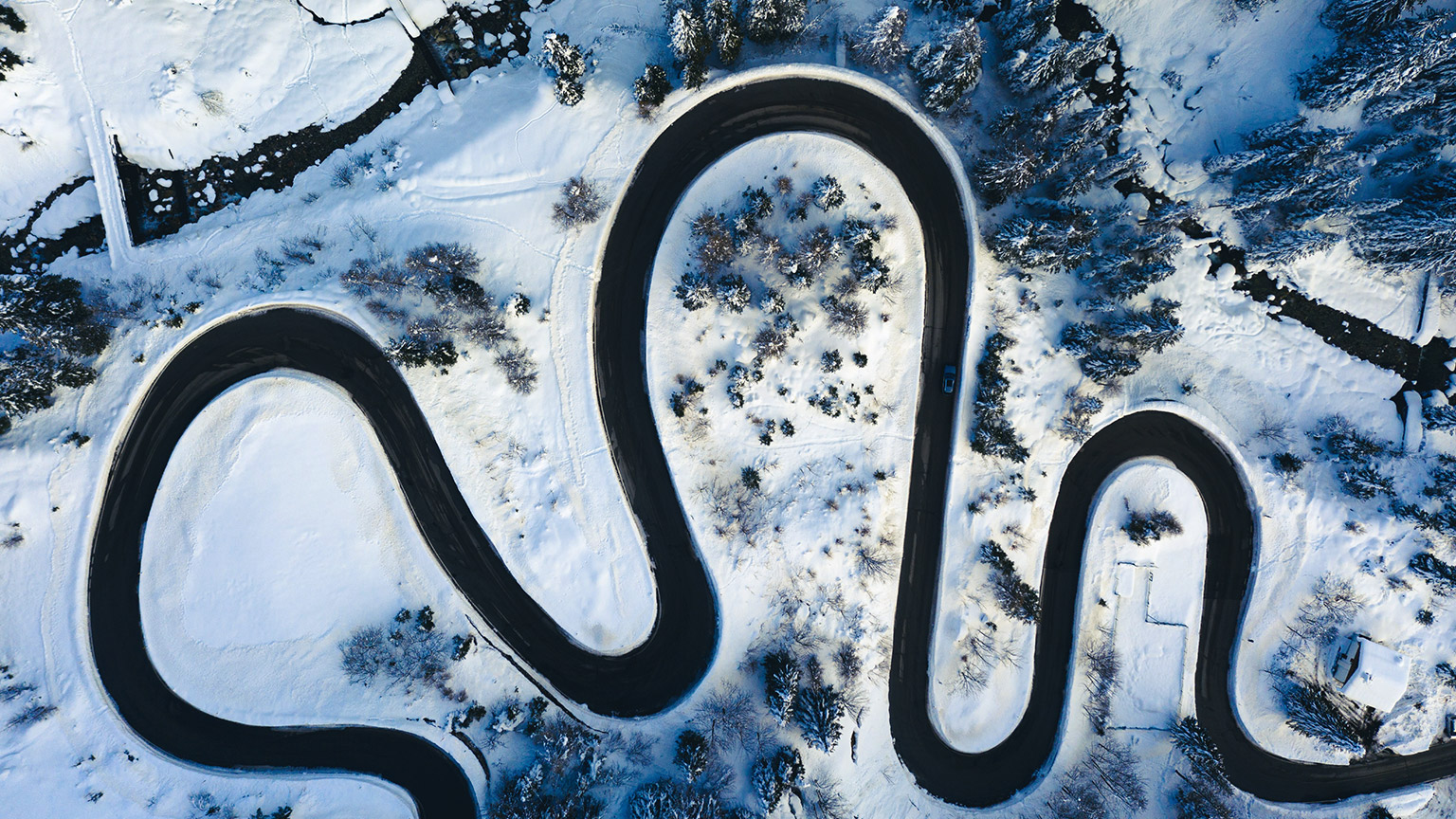